

- Home
- JavaScript
- How To Create Color Generator ...
Create Random Color Code Generator Mini-Projectย In HTML CSS and JavaScript
Building a Random color code generator in JavaScript could be a cool project! You could create a simple tool where users can Generate a color Code and User also can copy generated code. Users can Also copy Its Shades color and ย copy various formats (hex, RGB, HSL)
I have structured the code to generate a color palette with functions for creating elements, generating random colors, displaying color information, and handling various interactions like showing shades and copying color codes.
Output :
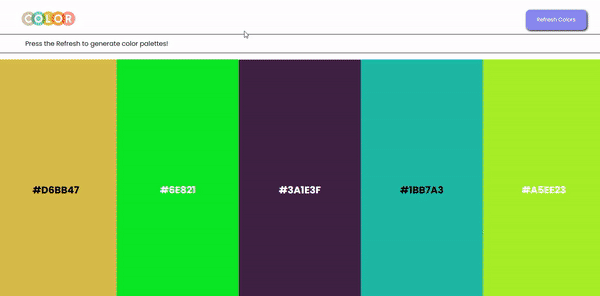
Let's Make Design Using HTML& CSS
First we need to create basic starting template with required links of CSSย links inย head section of HTML file.ย
Random Color Generator
Add HTML Elements Into DOM. Let’s make Structure.
Press the Refresh to generate color palettes!
Now Time to Move on CSS For Attractive Designย
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'poppins',sans-serif;
}
body {
width: 100%;
height: 100vh;
}
.navbar {
height: 20vh;
width: 100%;
display: flex;
justify-content: center;
flex-direction: column;
align-items: center;
}
.topnav, .options{
display: flex;
justify-content: space-between;
align-items: center;
width: 100%;
border-bottom: 1px solid black;
padding: 10px 3%;
}
.options{
padding: 10px 5%;
}
.view{
display: flex;
align-items: center;
gap: 10px;
cursor: pointer;
transition: all .5s ease-in-out;
border-radius: 10px;
padding: 10px;
}
.view:hover{
background-color: rgb(198, 198, 198);
}
.navbar button {
height: 50px;
width: 150px;
cursor: pointer;
color: white;
background-color: rgb(135, 135, 240);
border: none;
border-radius: 10px;
box-shadow: 2px 2px 5px black;
transition: all 0.5s ease-in-out;
}
button:hover{
box-shadow: none;
}
.container {
height: 80vh;
width: 100%;
margin: auto;
display: flex;
justify-content: center;
align-items: center;
flex-wrap: wrap;
}
.box {
height: 100%;
width: 20%;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
gap: 20px;
cursor: pointer;
}
.lightbox {
position: absolute;
width: 100%;
height: 100vh;
top: 0;
left: 0;
display: none;
background-color: rgba(0, 0, 0, 0.272);
backdrop-filter: blur(10px);
}
.lgbox_container {}
.lightbox .lgbox {
width: 50%;
background-color: aliceblue;
margin: auto;
margin-top: 10vh;
display: flex;
flex-direction: column;
}
.showlightbox {
display: block;
}
.lgshade {
height: 50px;
width: 100%;
display: flex;
justify-content: center;
align-items: center;
}
.lightbox i {
position: absolute;
top: 5%;
right: 10%;
font-size: 25px;
background-color: red;
height: 30px;
width: 30px;
border-radius: 10px;
display: flex;
justify-content: center;
align-items: center;
}
.iconbox{
display: flex;
gap: 20px;
opacity: 0;
transition: all .5s ease-in-out;
}
.box:hover .iconbox{
opacity: 1;
}
i{
font-size: 1.3rem;
}
.colorbox-color{
padding: 10px;
border: 1px solid black;
display: flex;
flex-direction: column;
gap: 20px;
}
.colorbox-color p{
margin: auto;
font-size: 20px;
}
JavaScript Code:ย
Now we need JavaScript code to implement color pallette features
let body = document.querySelector("body");
let button = document.querySelector("#btn");
let container = document.querySelector(".container");
let lightbox = document.querySelector("#lightbox")
let cancel = document.querySelector("#cancel")
//create DIV
let createDiv=(e)=>{
let div = document.createElement(e);
return div
}
//Random Number
let Numbgenrate=()=>{
return Math.floor(Math.random() * 255)
}
//Returns a Number Which is Red Color
let getRed=()=>{
let red = Numbgenrate();
return red
}
//Returns a Number Which is Blue Color
let getBlue=()=>{
let Blue = Numbgenrate();
return Blue
}
//Returns a Number Which is Green Color
let getGreen=()=>{
let Green = Numbgenrate();
return Green
}
let gethaxred=()=>{
let haxred = getRed().toString(16);
return haxred
}
let gethaxgreen=()=>{
let haxgreen = getGreen().toString(16);
return haxgreen
}
let gethaxblue=()=>{
let haxblue = getBlue().toString(16);
return haxblue
}
let RGBcolor=()=>{
return `${getRed()},${getBlue()},${getGreen()}`
}
let Hexacolor=()=>{
return `#${gethaxred()}${gethaxgreen()}${gethaxblue()}`
}
// View color code
let lgbox = document.querySelector("#lgbox");
function colorbox(a, text2) {
lgbox.innerHTML = "";
lightbox.classList.toggle("showlightbox");
// Create RGB color box
let rgbcolor = createDiv("div");
rgbcolor.classList.add("colorbox-color");
let titlergb = createDiv("h3");
titlergb.innerHTML = "RGB format :";
let textrgb = createDiv("p");
textrgb.innerText = `rgb(${a})`;
rgbcolor.append(titlergb);
rgbcolor.append(textrgb);
lgbox.append(rgbcolor);
// Create HEX color box
let hexcolor =createDiv("div");
hexcolor.classList.add("colorbox-color");
let titlehex = createDiv("h3");
titlehex.innerHTML = "HEX format :";
let texthex =createDiv("p");
texthex.innerHTML = text2;
hexcolor.append(titlehex);
hexcolor.append(texthex);
lgbox.append(hexcolor);
}
// shades code--------------------------------
function shades(a) {
lgbox.innerHTML=""
lightbox.classList.toggle("showlightbox")
for (i = 1; i <= 9; i++) {
let shade = document.createElement("div");
shade.classList.add("lgshade")
shade.style.backgroundColor = `rgba(${a},0.${i})`
let text3 = document.createElement("p")
shade.append(text3)
text3.innerHTML = `rgba(${a},0.${i})`
lgbox.append(shade)
shade.addEventListener("click", function () {
// alert(text3.innerHTML)
navigator.clipboard.writeText(text3.innerHTML);
showToast(`${text3.innerHTML} code copied!`);
})
}
}
// Main Function Which Is Execute
function colorpelette() {
container.innerHTML = "";
for (i = 1; i <= 5; i++) {
let box = createDiv("div");
let iconbox = createDiv("div");
let icon = createDiv("i");
let icon2 =createDiv("i");
let icon3 = createDiv("i");
iconbox.classList.add("iconbox");
icon.classList.add("fa-solid", "fa-border-all");
icon.setAttribute('title', 'View Shades');
iconbox.append(icon)
icon2.classList.add("fa-solid", "fa-copy");
icon2.setAttribute('title', 'Copy HEX Code');
iconbox.append(icon2)
icon3.classList.add("fa-solid", "fa-eye");
icon3.setAttribute('title', 'Color Codes');
iconbox.append(icon3)
icon.addEventListener("click", function () {
shades(text1.innerHTML)
})
icon3.addEventListener("click", function () {
colorbox(text1.innerHTML,text2.innerHTML)
})
icon2.addEventListener("click", function () {
navigator.clipboard.writeText(text2.innerHTML);
showToast(`${text2.innerHTML} code copied!`);
})
let text1 = createDiv("h2");
let text2 = createDiv("h2");
box.appendChild(text1)
box.appendChild(text2)
box.append(iconbox)
container.appendChild(box);
//RGB genrate here
let color = RGBcolor();
let bgcolor = `rgb(${color})`;
text1.innerHTML = color;
box.classList.add("box");
box.style.backgroundColor = bgcolor;
//HEXA Code genrate here
let colorhax = Hexacolor();
let colorhaxup = colorhax.toUpperCase();
text1.style.display="none"
text2.innerHTML = colorhaxup;
// if Backgorundcolor dark, Text Color Become white
if (getRed() < 50) {
if (getGreen() < 50) {
text2.style.color = "white";
iconbox.style.color = "white";
}
else {
if (getBlue() < 50) {
text2.style.color = "white";
iconbox.style.color = "white";
}
}
}
else{
if (getGreen() < 50) {
text2.style.color = "white";
iconbox.style.color = "white";
}
else {
if (getBlue() < 50) {
text2.style.color = "white";
iconbox.style.color = "white";
}
}
}
cancel.addEventListener("click",function(){
lightbox.classList.toggle("showlightbox")
})
}
}
function showToast(message) {
Toastify({
text: message,
duration: 2000, // Duration in milliseconds
close: true, // Whether to add a close button
gravity: "top", // toast position (e.g., "top-right", "top-center", "top-left", etc.)
position: 'right',
backgroundColor: "linear-gradient(to right, lightgreen, green)", // Set your desired background color
}).showToast();
}
// onload window
window.onload(colorpelette())
More Tutorials On YouTube Check Now:ย
- Personal Portfolio website Design In HTML CSS and JavaScript
- Animated Login Page
- Our Service Section Using HTML CSS and JavaScriptย
Recent Post
Page Titile Example Post25
- May 15, 2025
- 1 min read
Page Titile Example Post26
- May 15, 2025
- 1 min read
Page Titile Example Post27
- May 15, 2025
- 1 min read