

- Home
- JavaScript
- How To Make Digital Clock Java ...
Create Digital Clock Mini-Project In HTML CSS and JavaScript
A Digital Clock is a type of clock that displays the time digitally, usually using numbers to represent hours, minutes, and sometimes seconds.Unlike analog clocks, which use hands to indicate time based on the positions of the hour, minute, and second hands, digital clocks directly display the numeric time in a format like “HH:MM:SS” (hours, minutes, seconds).
Below Output :
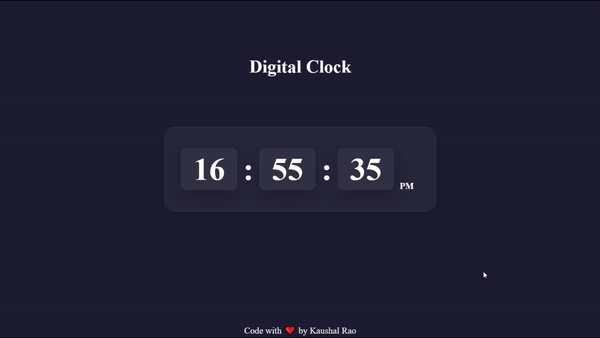
Let's Make Attractive Design Using HTML& CSS
First we need to create basic starting template with required links of CSS , CDN links in head section of HTML file.
Password Validation
Digital Clock
00
:
00
:
00
AM
Lets Move on CSS Code For Attractive Design
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: "poppins";
}
.heading {
color: white;
font-size: 2rem;
text-align: center;
position: absolute;
top: 0;
padding: 100px;
}
section {
position: absolute;
width: 100%;
height: 100vh;
background: #19172e;
display: flex;
justify-content: center;
align-items: center;
}
.clock {
position: relative;
width: 480px;
height: 150px;
background: rgba(255, 255, 255, 0.05);
box-shadow: 0 15px 25px rgba(0, 0, 0, 0.1);
z-index: 1000;
border-radius: 20px;
backdrop-filter: blur(20px);
}
.clock .container {
display: flex;
justify-content: center;
align-items: center;
height: 100%;
border:1px solid rgba(255,255,255,0.1);
border-radius: 15px;
}
.clock .container h2 {
font-size: 3.5rem;
color: #fff;
}
.clock .container h2:nth-child(odd) {
padding: 5px 15px;
width:100px;
text-align: center;
border-radius: 10px;
background: rgba(255, 255, 255, 0.05);
box-shadow: 0 15px 25px rgba(0, 0, 0, 0.2);
}
.dot{
padding:0px 10px;
}
#ampm {
position: relative;
top: 30px;
font-size: 1rem;
color: #fff;
font-weight: 700;
margin: 10px;
}
footer {
text-align: center;
color: white;
font-size: 1rem;
position: absolute;
left: 0;
right: 0;
bottom: 0;
padding: 5px;
line-height: 3vh;
}
footer a{
color:#f2f2f2;
text-decoration: none;
}
What Is 'Date' Object In Javascript?:
Date is the built-in-object .JavaScript’s Date object provides extensive functionalities to work with dates and times, allowing manipulation, comparison, and extraction of various components to suit different date-related needs in applications.
Getting Date Components:
You can get individual components of a date (year, month, day, etc.) using the get methods of the Date object
let date = new Date();
let year = date.getFullYear();
let month = date.getMonth(); // Month is 0-indexed (0 for January, 11 for December)
let day = date.getDate();
let hours = date.getHours();
let minutes = date.getMinutes();
let seconds = date.getSeconds();
What Is PedStart Method in Javascript ?:
The pedstart() method of string values pads this string with another string untill the resulting string reaches the given length .
Let's Move On Javascript Project :
const hour = document.getElementById("hour");
const minute = document.getElementById("minute");
const seconds = document.getElementById("seconds");
const ampm = document.getElementById("ampm");
// Add 0 to the begining of number if less than 10
function formatTime(time) {
return time.toString().padStart(2, "0");
}
function isAmPm(hours) {
return hours >= 12 ? "PM" : "AM";
}
function clock() {
const date = new Date();
let h = date.getHours();
let m = date.getMinutes();
let s = date.getSeconds();
hour.textContent = formatTime(h);
minute.textContent = formatTime(m);
seconds.textContent = formatTime(s);
ampm.textContent = isAmPm(h);
}
setInterval(clock, 1000);
More Tutorials On YouTube Check Now:
Recent Post
Var keyword in C#
- May 8, 2024
- 4 min read
What is Cloud Computing ? Definition &
- April 2, 2024
- 4 min read
Devika – An Open-Source AI Software Engineer
- March 27, 2024
- 3 min read