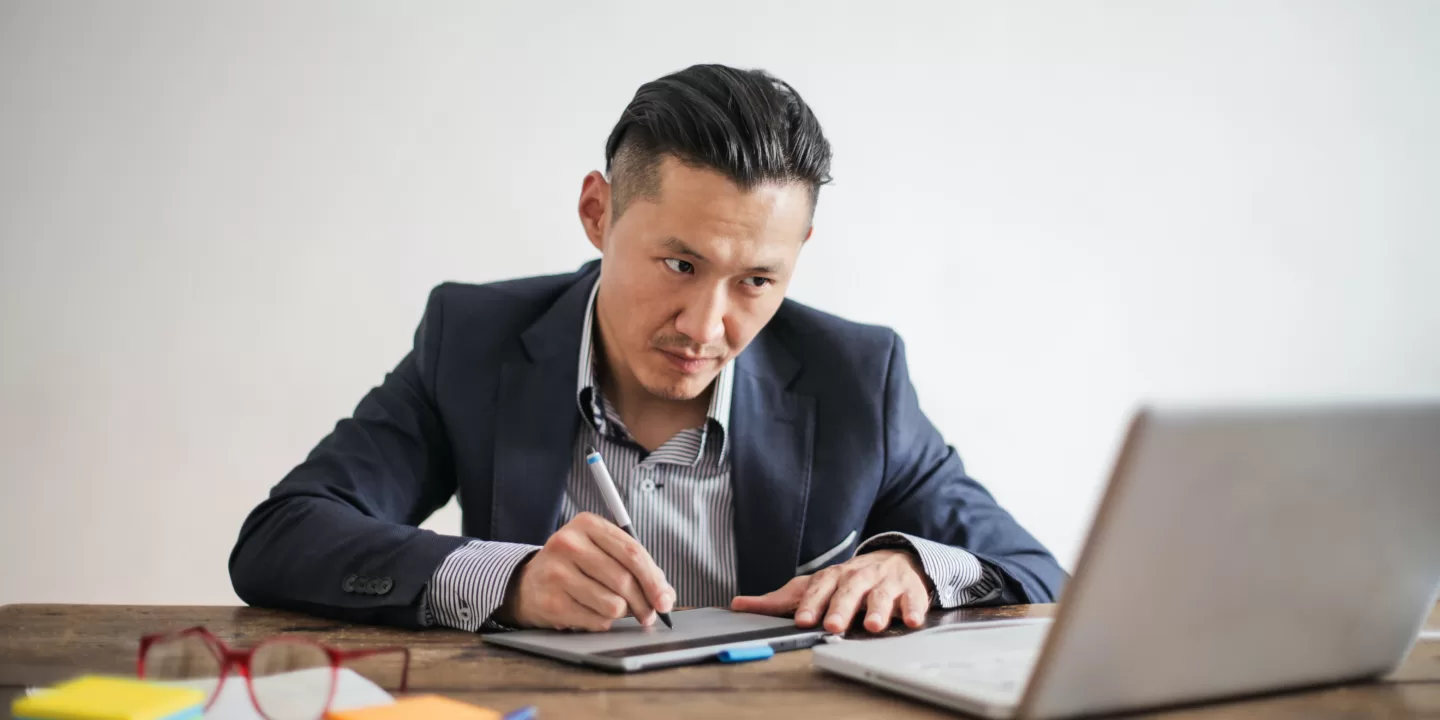
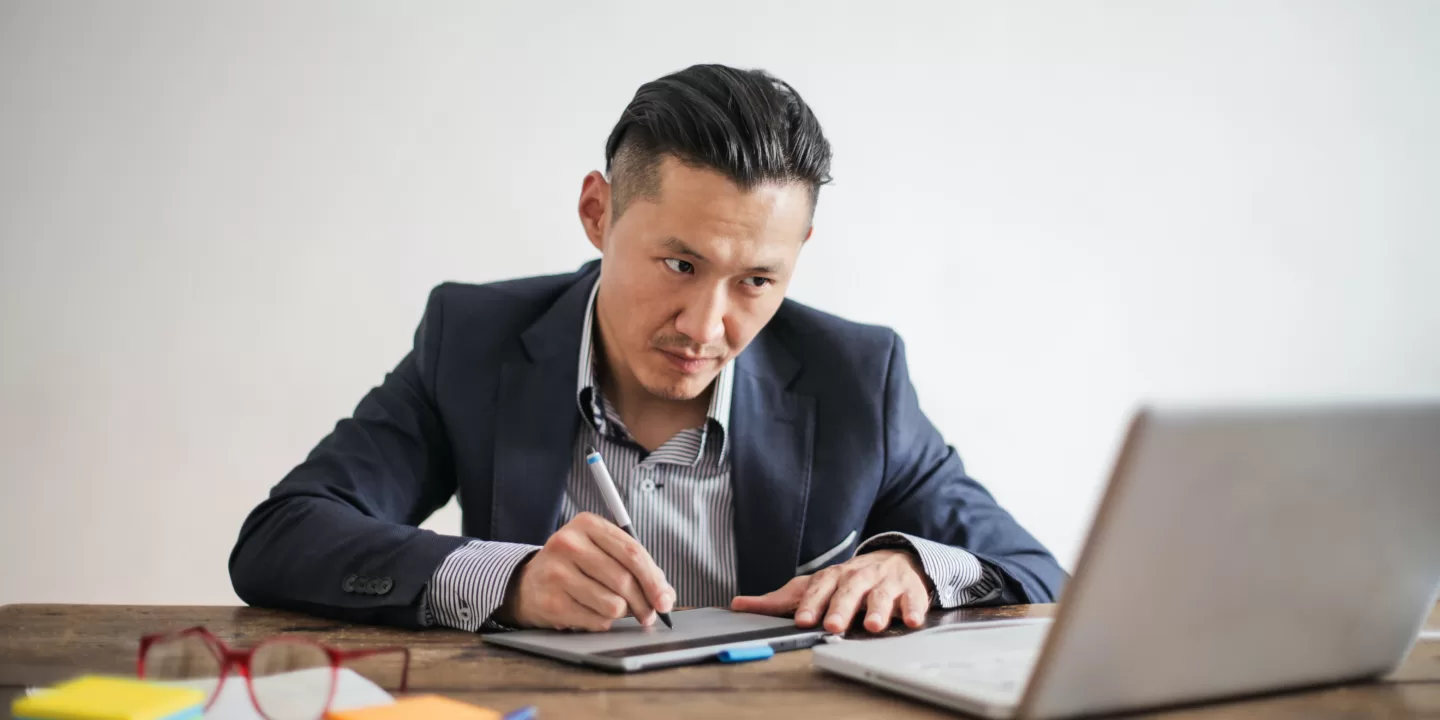
- Home
- JavaScript
- Template Literals In JavaScrip ...
Template literalsย or template stringsย allow you to use strings or embedded expressions in the form of a string. They are enclosed in backticks “. For example-
const name = 'Kaushal';
console.log(`Hello ${name}!`); // Hello Kaushal!
Without template literals, when you want to combine output from expressions with strings, you’d concatenate them using the addition operator +:
const a = 50;
const b = 100;
console.log("Total of " + a + " & "+b + "is : " +(a+b));
In the above example we usedย concatenate operator or addition operatorย +ย to evaluate expression with variable andย string, but sometime it becomesย moreย confusing or error proneย due to multiple double quotes, andย +ย (addition operators.)
With template literals, you can avoid the concatenation operator โ and improve the readability of your code โ by using placeholders of the form ${expression} to perform substitutions for embedded expressions:
const a = 50;
const b = 100;
console.log(`Total of ${a} & ${b} is : ${a+b}`;
Template Literals for Strings
In the earlier JavaScript you would use a single quote ” or a double quote “” for strings. But remember that we can not use same quote sign within the string means if you started a string with single quote cant useย single quote ‘ inside, or if you used ” double quoteย to start a string can not use same inside string, we can do this using escape sequence character ‘\’ For example,
const str1 = 'This is the first string';
// cannot use the same quotes
const str2 = 'A "quote" inside a string'; // valid code
const str3 = 'A 'quote' inside a string'; // Error
const str4 = "Another 'quote' inside a string"; // valid code
const str5 = "Another "quote" inside a string"; // Error
// escape characters using \
const str3 = 'A \'quote\' inside a string'; // valid code
const str5 = "Another \"quote\" inside a string"; // valid code
But template literals make above expression more easier without using escape sequence character.
// escape characters using \
const str3 = 'A \'quote\' inside a string'; // valid code
const str5 = "Another \"quote\" inside a string"; // valid code
Multiline Strings Using Template Literals
Template literals also make it easy to write multiline strings. For example,
// using the + operator
const message = 'This is a multiline message\n' +
'that spans across multiple lines\n' +
'in the code.'
console.log(message)
// using template literals
const message1 = `This is a multiline message
that spans across multiple lines
in the code.`
console.log(message1)
Expression Interpolation
Before JavaScript ES6, you would use the +ย ย operator to concatenate variables and expressions in a string.ย ย
With template literals, it’s really easier to include variables and expressions inside a string using theย ${...}
ย syntax.
The process of assigning variables and expressions inside the template literal is known as interpolation.ย For example,
//without template literals
const name = 'Kaushal'; console.log('Hello ' + name); // Hello Kaushal
// With template literals
const name = 'Kaushal';
console.log(`Hello ${name}`);
// template literals used with expression
const a = 50;
const b = 100;
console.log(`Total of ${a} & ${b} is : ${a+b}`;
Tagged Templates
Tagged templates behave like a JavaScript function. You use tags that allow you to parse template literals with a function.
Tagged template is written like a function definition. However, you do not pass parentheses () when calling the literal. For example- first we create a simple function with parameters, and then demonstrate tagged templates
// its just normal function with parameter only
function tagTemplate(strings) {
return strings;
}
// passing argument
const result = tagTemplate('Kaushal Rao');
console.log(result);
// creating tagged template
const result = taggedTemplate`Kaushal Rao`;
console.log(result);
// output - ite returns an array
["kaushal Rao"]
Recent Post
Top 5 Login & Registration Form Using
- April 9, 2025
- 3 min read
Modern Registration Form using HTML, CSS &
- April 9, 2025
- 5 min read
Simple Registration Form Using HTML & CSS
- April 9, 2025
- 4 min read