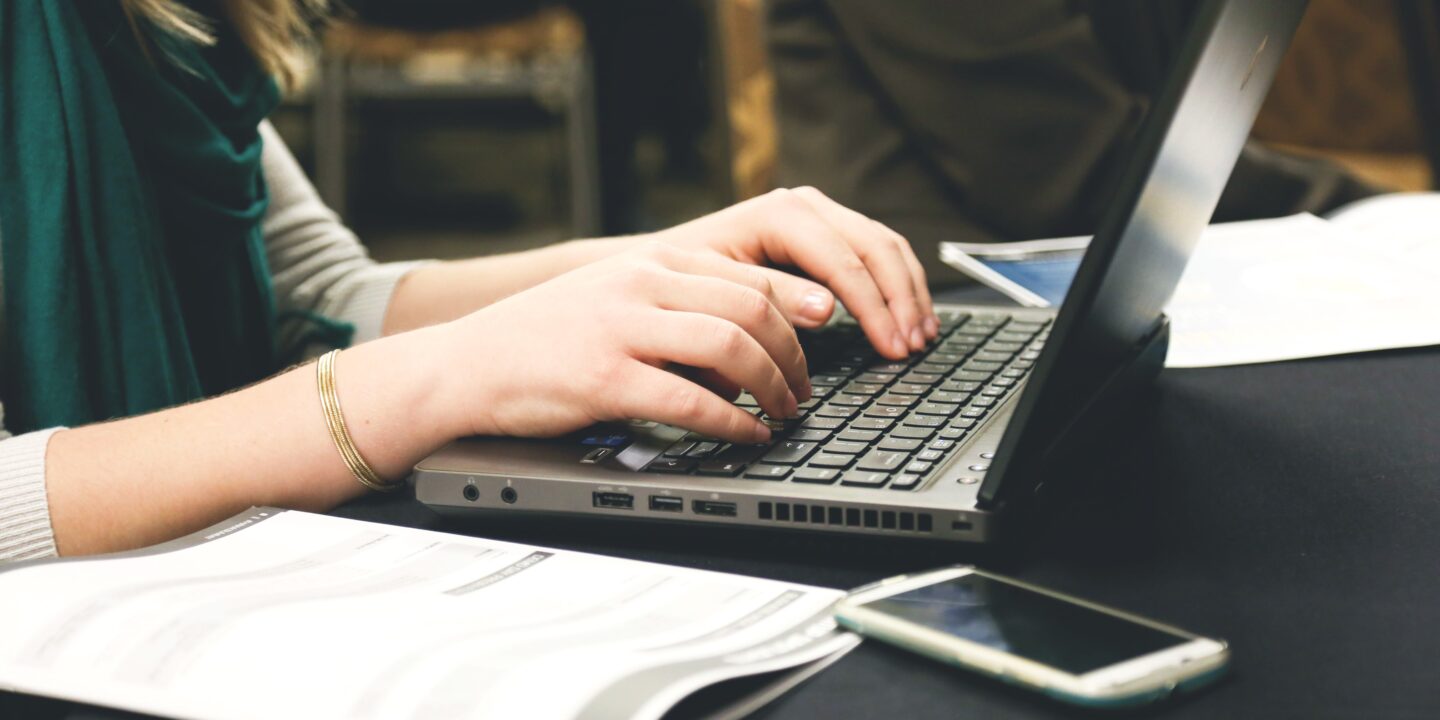
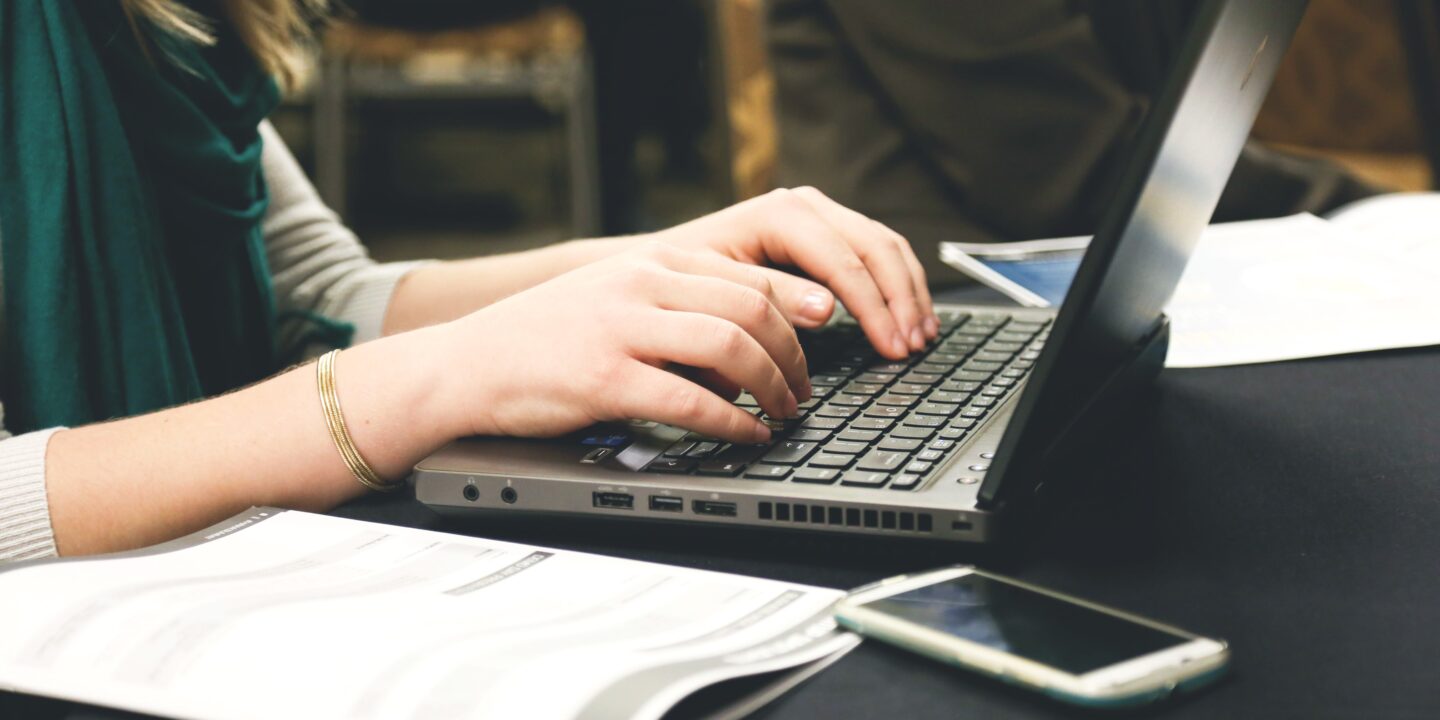
- Home
- JavaScript
- Document Object Model , What i ...
Introduction to JavaScript DOM
In JavaScript, the DOM stands for the Document Object Model. It is a programming interface for web documents. When a web page is loaded in a browser, the browser creates a representation of the document in memory, which the script can access and manipulate. This document structure forms a tree-like model where each element of the document, like paragraphs, headers, images, forms, etc., is an object in the tree. These objects can be accessed, manipulated, and modified using JavaScript.
The DOM allows JavaScript to interact with the web page. It enables developers to dynamically access and modify the content, structure, and style of the document. For example, JavaScript can be used to:
- Access and modify HTML elements and their attributes.
- Add or remove HTML elements.
- Change the content or style of a webpage.
- Handle events like clicks, mouse movements, etc.
- Manipulate CSS styles and classes.
For instance, with JavaScript, you can select an HTML element, change its content, style, or even create new elements and place them within the document—all by utilizing the DOM API methods and properties. It works on HTML elements for getting, changing, adding, or deleting HTML elements. With the help of JavaScript code along with HTML DOM, one can able to change the content of HTML elements.
The DOM represents an HTML document as a tree of nodes. The DOM provides functions that allow you to add, remove, and modify parts of the document effectively.
Note that the DOM is cross-platform and language-independent ways of manipulating HTML and XML documents.
DOM - Hierarchy of nodes
The DOM represents an HTML document as a hierarchy of nodes. Consider the following HTML document:
JavaScript DOM
Hello DOM!
The following tree represents the above HTML document:
In this DOM tree, the document is the root node. The root node has one child node which is the <html> element. The <html> element is called the document element.
Each document can have only one document element. In an HTML document, the document element is the <html> element. Each markup can be represented by a node in the tree.
JavaScript DOM is worked as a programming interface for HTML as well as for XML documents. This works with its related pages so HTML code can change things like structure, content, and style too.
DOM along with JavaScript helps to access all kinds of elements in the concern of the webpage. The DOM of the webpage is created whenever the webpage is getting loaded into the system. It is created in the system like a tree structure.
With the help of DOM along with JavaScript, one may be able to do multiple things at a time those are like Working with attributes for different functionalities, creating new attributes and elements, changing already presented attributes and elements, removing the already available attribute and element, to create a new event on a page with the help of attributes and elements and many more exciting tasks one can do using this methodology.
One can define a logical structure as well as defining ways to access and manipulate documents using DOM. DOM doesn’t work as a binary description means it doesn’t describe any kind of binary source code within its respected interface.
It helps to perform tasks like finding elements, changing the document, creating nodes, dealing and working with attributes, Designing and working with layouts, styling to the particular attribute or elements which are available in HTML page, it also works with cascading stylesheet, working with query selectors, positioning and animating with the help of JavaScript using DOM and much more interesting thing you can do using JavaScript DOM.
Hierarchical Structure
In JavaScript, the Document Object Model (DOM) represents a web page as a hierarchical structure, much like a tree. This hierarchy is composed of elements, attributes, and text, organized in a tree-like fashion, with a single top-level node called the “document.” Here’s an explanation of this hierarchical structure in the DOM:
Window Object: The window object is treated as a parent object to all of the objects. So all other objects are treated as child object in this hierarchy. The window object is known as a top-level object for each other objects. It includes properties methods like closed, document, name, status, self, location, etc.
Document Node: The top-level node in the DOM hierarchy is the document node. It represents the entire web page. You can access it using document in JavaScript.
Elements: Elements are the building blocks of a web page, represented by nodes in the DOM. They include HTML tags like <div>, <p>, <h1>, and others. Elements can contain other elements, forming a parent-child relationship. For example, a <div> element can contain multiple <p> elements as children.
Attributes: Elements can have attributes, such as id, class, and src. These attributes are also represented as nodes in the DOM hierarchy and are attached to their respective elements.
Text Nodes: Text nodes represent the text content within elements. For example, the text within a <p> element is stored in a text node. Text nodes are child nodes of their respective elements.
Parent-Child Relationships: Elements and nodes in the DOM have parent-child relationships. For example, a <div> element can be the parent of multiple <p> elements, and each <p> element is a child of the <div>. This hierarchical structure allows you to traverse the DOM and access specific elements and their contents.
Siblings: Elements that share the same parent are called siblings. Siblings are nodes that are at the same level in the DOM hierarchy. You can access and manipulate siblings using JavaScript.
Traversal: You can traverse the DOM hierarchy using various JavaScript methods, such as querySelector, getElementById, and nextSibling. These methods help you navigate from one node to another within the DOM tree.
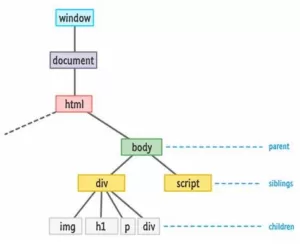

For detail video –
Recent Post
Var keyword in C#
- May 8, 2024
- 4 min read
What is Cloud Computing ? Definition &
- April 2, 2024
- 4 min read
Devika – An Open-Source AI Software Engineer
- March 27, 2024
- 3 min read