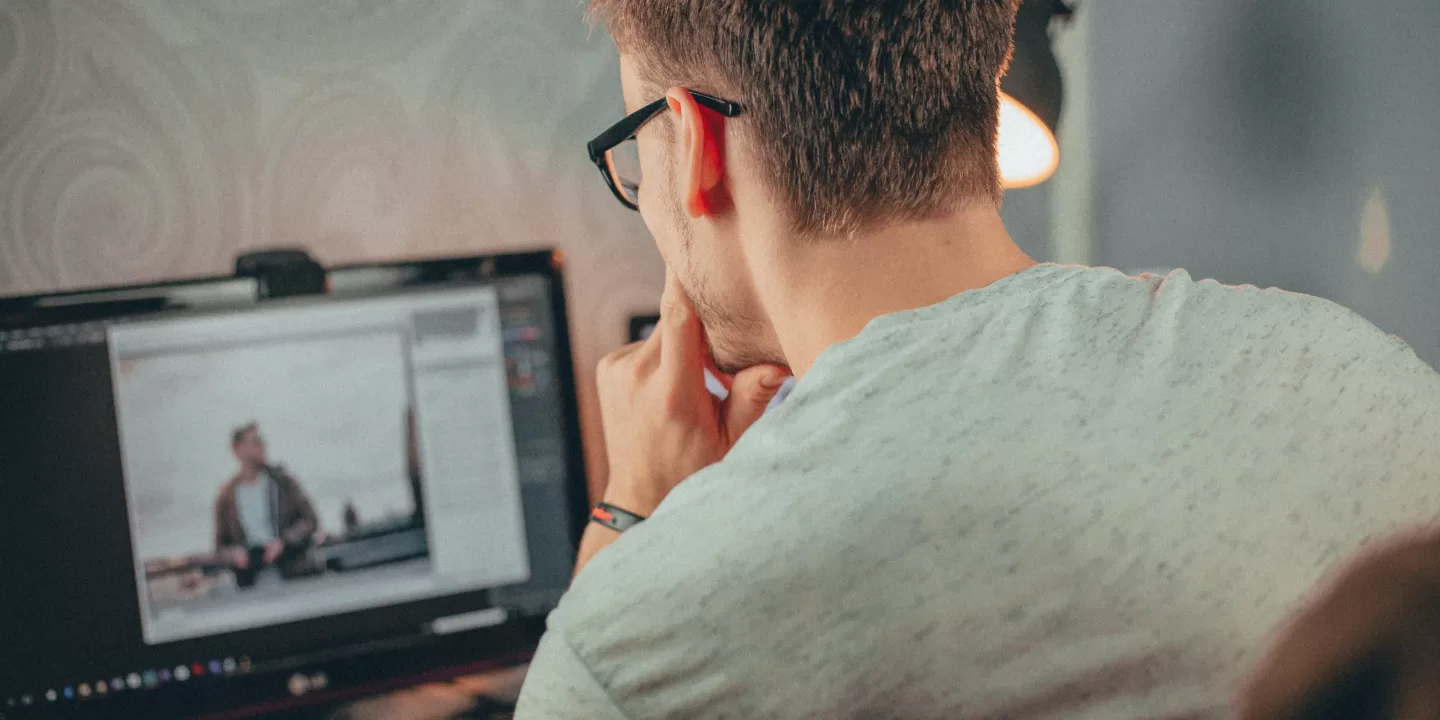
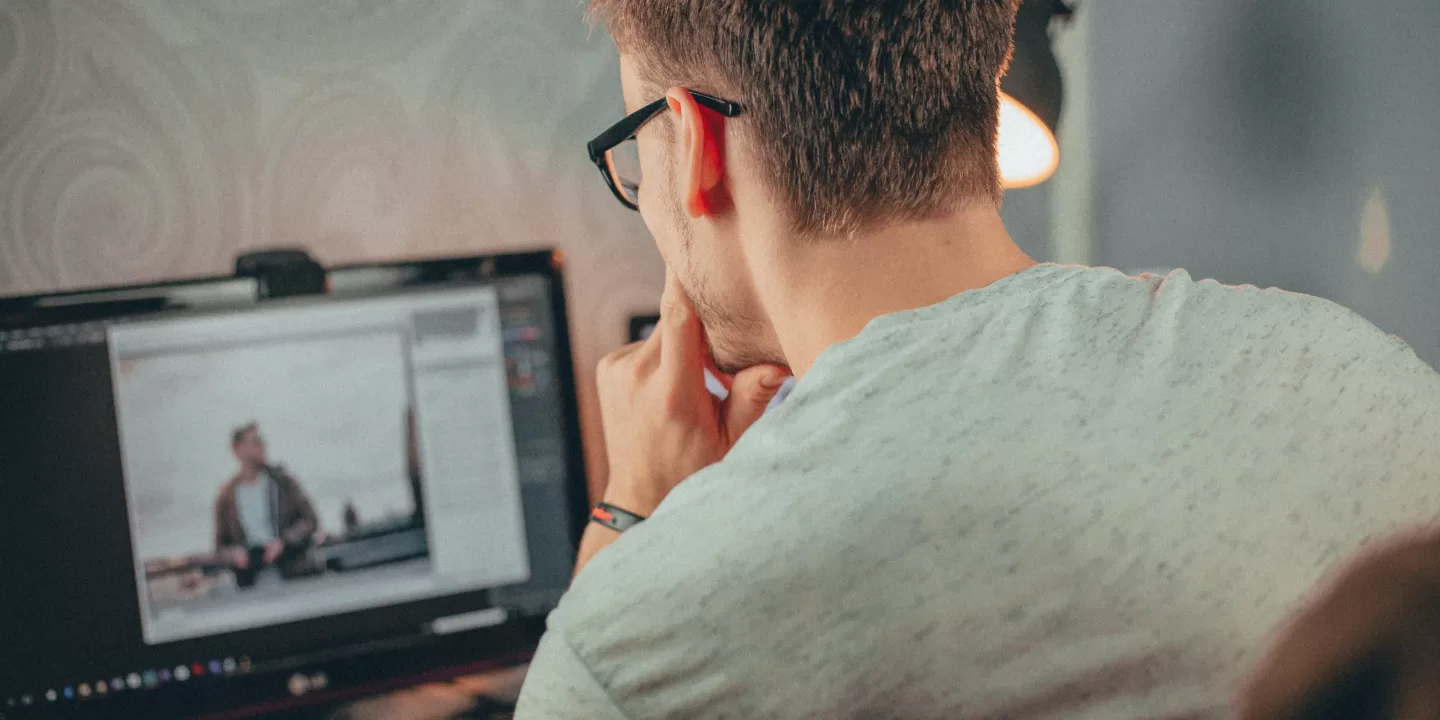
- Home
- JavaScript
- JavaScript Scope
What is scope in Javascript
Scope in JavaScript refers to the accessibility or visibility of variables and expressions. That means the space where an item, such as a variable or a function, is visible and accessible in your code.
For example, once a variable is declared, it can only be accessible within the scope that it has been declared in and will not be accessible outside the scope.
JavaScript has 3 types of scope:
Global scope Block scope/Function scope Local scope
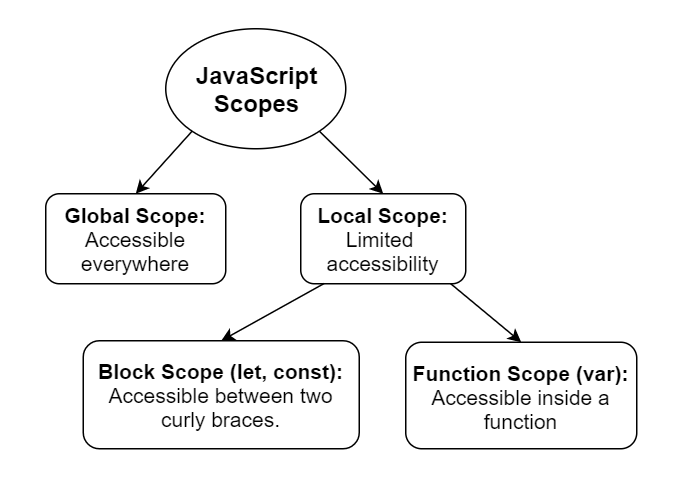
Global Scope
let Name = "Kaushal";
// code here can use Name
function myFunction() {
// code here can also use Name
}
Block scope
The scope created with a pair of curly braces (a block).
A function creates a scope, so that (for example) a variable defined exclusively within the function cannot be accessed from outside the function or within other functions. For instance, the following is invalid:
function exampleFunction() {
const x = "declared inside function"; // x can only be used in exampleFunction
console.log("Inside function");
console.log(x);
}
console.log(x); // Causes error
const x = "declared outside function";
exampleFunction();
function exampleFunction() {
console.log("Inside function");
console.log(x);
}
console.log("Outside function");
console.log(x);
Local Scope
Variables declared within a function are only accessible from within that function. This is known as function scope. Function scope helps to prevent variables from conflicting with each other and makes your code more modular and reusable.
// code here can NOT use Name
function myFunction() {
let Name = "Kaushal";
// code here CAN use Name
}
// code here can NOT use Name
Recent Post
Var keyword in C#
- May 8, 2024
- 4 min read
What is Cloud Computing ? Definition &
- April 2, 2024
- 4 min read
Devika – An Open-Source AI Software Engineer
- March 27, 2024
- 3 min read